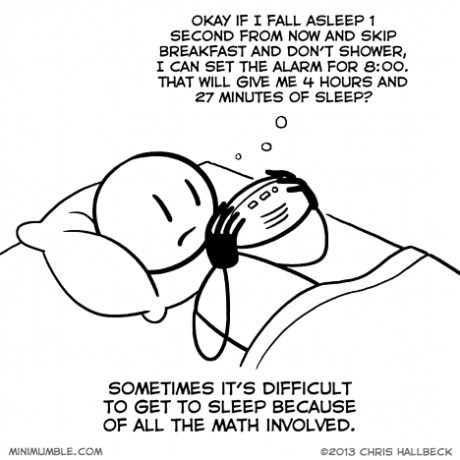
Mathematics made computer science possible. From the Turing-Church papers to Von Neumann architecture, many of the things we take for granted are the direct result of some mathematician’s discoveries.
University computer science programs require students to take math courses. To some extent, this is a reasonable requirement. Learning about DFAs and NFAs are the underpinning of creating compilers. Learning about relations is important in understanding database design. Clearly, mathematics has had a profound influence over computer science.
The irony is that only a small fraction of computer science graduates utilize the broad span of discrete mathematics and computational theory out in the workforce. So why are universities so set on requiring these courses to computer science students? Is it to discourage those “on the fence” about their area of study? Is it just that tradition is difficult to break? Or are these courses truly important (and if so, for who?)?
The Mathematics Experience
There are many reasons students struggle in the mathematics courses universities require for a degree in computer science. For one, American students generally come from schools that teach math in a rather ineffective way, as shown through numerous studies (the US Department of Education disputes this…imagine that). When students arrive in their Mathematical Foundations of Computer Science and Theory of Computation courses, they’re ill prepared to handle the concepts being covered. This is especially true if they were not able to take higher level mathematics courses in high school.
In addition, as with most college courses, the material is taught in a “one size fits all” manner. The expectation is that students be responsible for their own learning, utilize recitations and office hours to ask questions, and find supplemental material to help them learn as needed. Unfortunately, a large portion of the mathematical concepts in computer science are so abstract that no matter what book, website, or video a student finds, the material is presented in exactly the same way as it was taught in class. If that method isn’t helpful, there are no other recourses.
Finally, there’s the problem of motivation. Students know they will not be writing proofs, drawing DFAs, or calculating the closure of a set of functional dependencies out in the workforce. For some students, these things may be exciting and worthwhile, but for others, learning these concepts is a waste of time. They take the course because it is a requirement, not because they are truly interested in the material or think it will be beneficial to their future career. And what happens when the course is over? ALL IS FORGOTTEN.
The Push For Mathematical Theory in Computer Science
Required mathematics courses may be lacking in practicality, but many computer science courses can also become bogged down in mathematical theory. Take, for instance, the topic of databases. Relational databases surely have their roots in relational algebra and calculus. To create a well-designed database, one must understand functional dependencies, closure, and algorithms for such things as BCNF. Yet, these concepts are mathematically intensive and for the average computer science student, they are completely unnecessary for learning how to work with the databases they will encounter after they graduate. The time invested in teaching mathematical theory takes away time to teach other, more practical skills and concepts in computer science.
A Modest Proposal
I propose that there should be two tracks of computer science. During a student’s freshman year, they take courses that give a general overview of different areas of study within the field of computer science. This way, they are informed about the different careers available to them, from DBA to data scientist to front-end developer.
In the sophomore year, they begin to branch into one of two tracks. The first is more mathematically intensive and for good reason - their career paths require it. If a student wants to do machine learning, they need to have a good grasp of statistics, linear algebra, and calculus to write the code. It’s really just that simple. There are certain areas of computer science that require a high level of mathematical prowess. This track is for students who wish to pursue those areas.
The other track is less concerned about mathematics. It still contains math coursework and in some cases, integrates mathematics into its computer science courses. The difference is that it teaches very practical math that is actually used to do specific tasks in computer science. Theoretical mathematics is left to the first track, since those students will benefit more from learning it. The students in this track may learn some of the same concepts as their peers in the first track, but in a more concrete way. For example, a student in this track may learn about relational databases and how lossless join works, but it will be taught using plain language, not in mathematical notation.
What about graduate school, you may be wondering. I still propose that there should be two options for certain courses, like Databases and Machine Learning. The University of Pennsylvania offered two machine learning courses at the graduate level this semester: one which was math intensive, one that was less so. Machine learning is so in demand by industry right now that it just makes sense to provide multiple ways of teaching the material to maximize the amount of people who understand and practice it. Clearly stripping away some of the mathematics in computer science courses can be done with great success and provide access to concepts that used to be reserved for the mathematically-inclined.
You may think I’m advocating for dumbing down computer science by removing mathematics. I’m not. What I am advocating for is making computer science coursework more tailored to the needs of both students and industry. It’s time to reevaluate what is being taught in computer science courses and make room to teach what is truly important today and in the years to come.